|
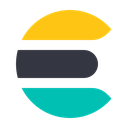
Elastic Cheat Sheet
Elasticsearch is a search engine based on the Lucene library. It provides a distributed, multitenant-capable full-text search engine with an HTTP web interface and schema-free JSON documents.
Elastic General
Cardinality deduplication statistics
Statistics grouped by multiple filters, grouped by filter
|
Terms group by field
|
bool combined query
|
Compound query
must |
子句必须在文档中匹配。 正匹配有助于提高相关性分数。 |
should |
不强制要求必须匹配。 但是,如果匹配,相关性得分就会提高。 |
must_not |
条件不得与文档匹配。 该子句不会对分数做出贡献(它在过滤上下文执行上下文中运行) |
filter |
条件必须与文档匹配,类似于 must 子句。 该子句不会对分数做出贡献 (它在过滤上下文执行上下文中运行) |
_msearch searches multiple indexes simultaneously
|
Batch query based on ID (search multiple indexes at the same time)
|
match_phrase strict match
|
range range query
|
simple_query_string simple version of combined keywords
|
match split words, sort by score after matching
|
- 先对关键字进行分词,然后按分词进行查询
- 按匹配度从大到小排序
- 分词之前默认匹配关系为or
- 按匹配度从大到小排序
- 分词之前默认匹配关系为or
multi_match match multiple fields
|
ids single index, matching multiple IDs
|
fuzzy query
|
- 允许不完全匹配,比如错别字,多字,少字
- fuzziness:设置误差范围,允许0-2之间
- fuzziness:设置误差范围,允许0-2之间
wildcard query
|
query_string Combining keywords
|
Term does not segment words, matches keyword type
|